Denial. Anger. Bargaining. Depression. Acceptance… Yes, my reader, this is not your Monday morning. There is something worse.
BUGS
May the souls of the broken keyboards and cracked monitors rest in peace because it’s hard to explain the cocktail of emotion that hits your poor soul at 2AM while searching for that one semicolon that throws an error on line 132 (but your file has only 50 lines).
But it doesn’t always have to be like this. There are a lot of habits and techniques that can save your brain cells for the next project. In this guide, we will look over the practical that every developer wished he knew before starting to code.
- Get used to writing clean code
- 1. Variable and function names have to talk for themselves
- 2. Most don’t care how you write the function's brackets but be consistent
- 3. Don’t be stingy with tabs and whitespace
- 4. Tabs or spaces?
- 5. Declare ALL your variables at the beginning of a function
- 6. No more than 3 variables as input to your function
- 7. Commenting your code
- 8. Are your functions too long?
- Use version control
- Try debugging yourself
- Consider taking another path
- Conclusion
Get used to writing clean code
Wanna see the best code I’ve ever written?
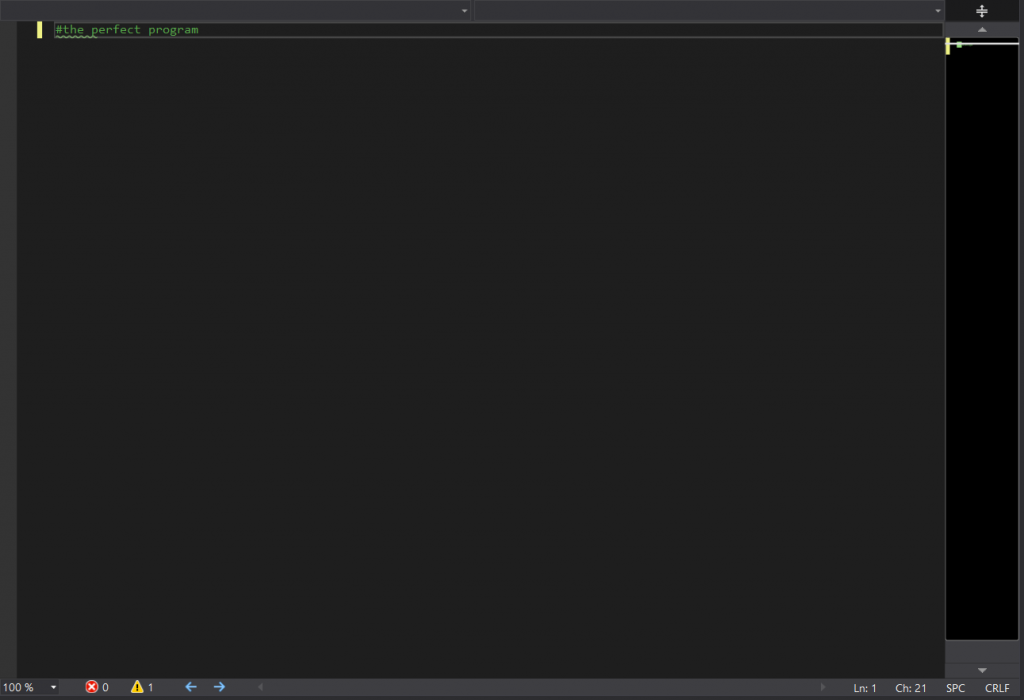
Jokes aside, I remember as a beginner being impressed by the amount of code a program had (we have all been there, right?). As counter-intuitive as it may sound, you want your application to have the least amount of code possible. The less code you have, the lower are the chances of something going badly.
Almost all (if not all) languages have a comprehensive style guide which is a great starting point if you are on a team that has a hard time reading each other’s code. These style guides go into much detail about recommended naming schemes, declarations, function/file lengths and many more.
Just google: “[Programming language name] style guide” and have a look.
For the “TLDR-people” reading this, here are the main changes you can make to increase the readability of your code today.
But before we start, note that there are 4 main naming styles in programming:
snake_case – words delimited by an underscore.
PascalCase – words delimited by capital letters.
camelCase – words delimited by capital letters except for the first word.
sHungarianNotation – camelCase but the first word/letter tells the variable type (“s” meaning string in this case) or intent of the function.
I started with snake_case because it is the preferred style in C but Python, for example, recommends a combination of the camel and Pascal cases. As you can see it varies from language to language. For this guide, we’ll stick with camelCase and Pyhton.
1. Variable and function names have to talk for themselves
int t #time elapsed from game start
Or
int tElapsed #from game start
Becomes
int timeElapsedGameStart
This way you don’t have to explain what the variable stores and there is no confusion.
“But Dan, such long names take more time to type!”
Well, get over it. A lot of code editors have auto-complete and you’ll thank me when you won’t need to scroll up to find the comment for your “t” variable you came up with last month.
2. Most don’t care how you write the function’s brackets but be consistent
Python does not have this issue but most other languages have this decade long debate.
In C++, for example, it’s either:
int numberSum(int left, int right) {
return 0;
}
Or
int numberSum(int left, int right)
{
return 0;
}
Tip: If you are on a team of Java developers go with the first one and don’t ask any questions. Thank me later😉
3. Don’t be stingy with tabs and whitespace
Again, an issue less prevalent in Python but some of this is applicable.
C++ is a great example:
int numberSumAndDouble(int left,int right) {int sum; sum=left+right; sum=sum*2; return sum}
Becomes
int numberSumAndDouble(int left, int right)
{
int sum;
int sumDouble;
sum = left + right;
sumDouble = sum * 2;
return sum;
}
Both examples will compile properly but the second one is much easier to read.
Variable declarations are separated from blocks of code and return statements are separated from everything else.
Space is added after commas between variables.
Variable declarations are properly lined up with spaces or preferably tabs.
Spaces are put around assignments and arithmetic operations.
4. Tabs or spaces?
I am a tabs advocate. Put your pitchforks down. I am not here to convince you which way to indentate your code.
You have a choice between 3 paces, 4 spaces and a tab. Pick one and ALWAYS use that one method. Change it only if your teammates have issues reading your code after copy-pasting it a couple times.
5. Declare ALL your variables at the beginning of a function
As you saw above, variable “sum” is first declared in a separate block and only then used.
Don’t
int sum = left + right;
For-loops are the only exception. The iterator is initialized when used:
int forLoopSum(int left, int right)
{
int sum;
for (index = 1; index <= 4; index++)
{
sum = sum + left;
sum = sum + right;
}
return sum;
}
Yeah, I know this function is weird, but it shows that “index” does not have to be declared in advance.
6. No more than 3 variables as input to your function
Don’t
int sumNumbers(int num1, int num2, int num3, float num4)
If you need more than 3 variables as input consider grouping them in data structures like lists, dictionaries, arrays, pairs, etc.
7. Commenting your code
This part is really important. If you ever come back to your old code you will never recall the entirety of what your code does. In a few years of coding, you will end up with a code dump like me. Don’t be like me, start commenting your code.
Only variable declarations can get an in-line comment like this:
int sum #the sum of numbers
Functions, classes, loops and anything in between get a comment on the line above like this:
#Traversing string and printing each letter twice
for letter in inputString:
print(letter)
print(letter)
As you can see, the comment makes sense here only because I am doing something unexpected with the string that need explaining. If I just wanted to traverse the string and print each letter, the comment would be pretty useless, as it would be easy to guess my intentions.
8. Are your functions too long?
In the Bootcamp I participated in, we had strict rules even to the number of lines a function could have (25) and the number of functions a file could have (3). These rules drove people crazy but enforced some good practices in the long run.
You don’t have to torture yourself by imposing these limitations but be mindful that if your code gets pretty long, think of breaking it up in smaller functions (even 2-5 lines long).
Making operations and writing edge cases separately is always a good idea.
for letter in inputString:
if letter == ‘.’ or letter == ‘?’ or letter == ‘!’:
break
else:
print(letter)
Becomes
def isEndOfLine(letter):
if letter == ‘.’:
return True
elif letter == ‘?’:
return True
elif letter == ‘!’:
return True
else:
return False
for letter in inputString:
if isEndOfLIne(letter):
break
else:
print(letter)
Yes, the code is much longer but each case is now clearly visible and easy to isolate when debugging. With longer codebases, this becomes crucial and a huge help!
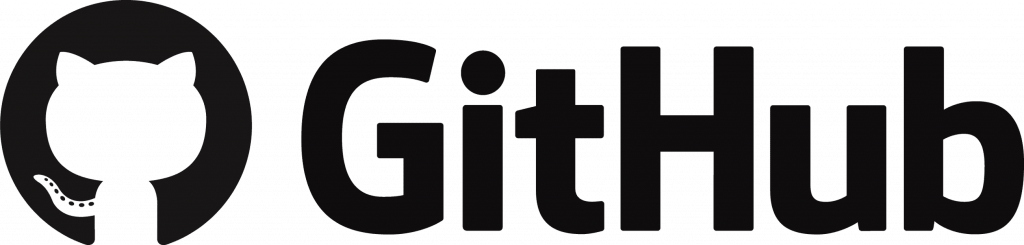
Use version control
Wait, wait! Stop scrolling, it is not as bad as it sounds. It is easy to get started with a version control system like GitHub and it saves a lot of nerves down the line.
You will no longer have to stress about losing your work or moving your work to another computer. Also, if your code goes “Nuke mode” (almost always does) you can always go back to the last stable version.
To get started, go to GitHub.com, make an account and create a repository (the folder that will contain your code). Now, depending on what operating system you are using, install GitHub on your computer.
I recommend getting comfortable with the terminal when working with git but other interfaces are good enough, to begin with. You can test that everything works fine by typing “git” in your terminal. If there is no “Command not found” you are good to go.
After the initial setup (it should take 10-15min the first time), you are now officially a citizen of the open-source community.
It’s time to get your code out there and save it in the cloud in a few simple steps.
First things first…
MAKE SURE YOU ARE OUTSIDE THE FOLDER OF THE PROJECT YOU WANT TO UPLOAD.
I can’t count the number of times I got confused by cloning repositories in the project folder with the same name.
1. Clone your repository
1.1. Find your repository’s main page, if you are not there yet, at the address: https://github.com/[your GitHub username]/[repository name]
1.2. Copy the address of your repository which should look like the link above but with a “.git” at the end.
1.3. In your terminal type:
git clone [the link you copied in the previous step]
If everything worked, you now have a message saying that you created an empty repository and an empty folder created with the name of your repository. You can make sure that it is the folder of your repository by going in it and typing:
ls -la

The output should have a line containing the hidden folder .git (The other files here are irrelevant).
2. Put something in the cloned repository
Copy the files you want to track or save in the created directory or start working in it.
Remember that only the files and documents in the repository will be tracked!
3. Submit your changes
3.1. Go to the root directory (the one that has the .git file)
3.2. Update the contents of all files to git with:
git add *
3.3. Record the changes to your project files with:
git commit -m “[A name for the changes made]”
3.4. Submit the changes:
git push
The files of your project should be now visible in your repository’s web interface.
Congratulations! Now your files are safely stored with git and the changes are recorded. Every time you make some significant changes to your project make a checkpoint by doing Step 3. In a few months, updating the repository becomes second nature.
Reverting to a previous state is a bit trickier but there is a great StackOverflow answer that explains the process step by step.
Try debugging yourself
How do you think others find the bugs in your code? More experienced developers can find bugs faster, no surprise. But you can tap into that by learning a couple tricks.
1. Print the values of your variables
Perhaps the easiest and one of the first methods used when confused with the output. Pick a line in your code where you suspect the bug occurs and print the output at that point in the logic.
Avoid printing in long for-loops. The vast amount of data it prints out just slows you down. Try to have just a few lines of code as output.
Don’t abuse this method. Printing all the variables in different places at the same time just creates a confusing wall of text. Also, expect some weird outputs if you are not sure what you are printing can be properly displayed as a string.
If you want to test the logic just print a string in the if statement brackets.
Tip: Use a distinct string that pops up. I prefer print(“wtf”).
2. Use a debugger
This is the fancy method, usually reserved for the “bourgeoisie” using IDEs. Instead of printing to the console, you can track the variable values while the program runs step by step up to a breaking point you set.
It is one of the most efficient and straight forward methods that checks if your code does what you intended. Learning your IDE’s debugger will be an incredible time investment you won’t regret.
3. Comment your bug out
A good method to use if your code is so broken it doesn’t even compile properly.
If you got a lot of functions calling other functions, you can start narrowing it down by commenting out certain function calls in order.
For a more radical solution, you can even start with entire blocks of code at a time and then go small. Once you find the bad block you can start looking through its logic and use the other methods to narrow down the exact issue.
4. Use try/catch
More of an error handling functionality but it can be used for debugging errors that make an app crash without an output.
Try/catch can be used to isolate suspicious blocks of code. When an error is thrown inside the try part, instead of crashing, the app executes the catch part and moves on. Just wrap the code in the “try” part and add a print statement in the catch part like this (Python):
try:
someBlockOfCode()
andSomeMore()
except:
print(“error”)
This way you can safely find the exact line that breaks your program.
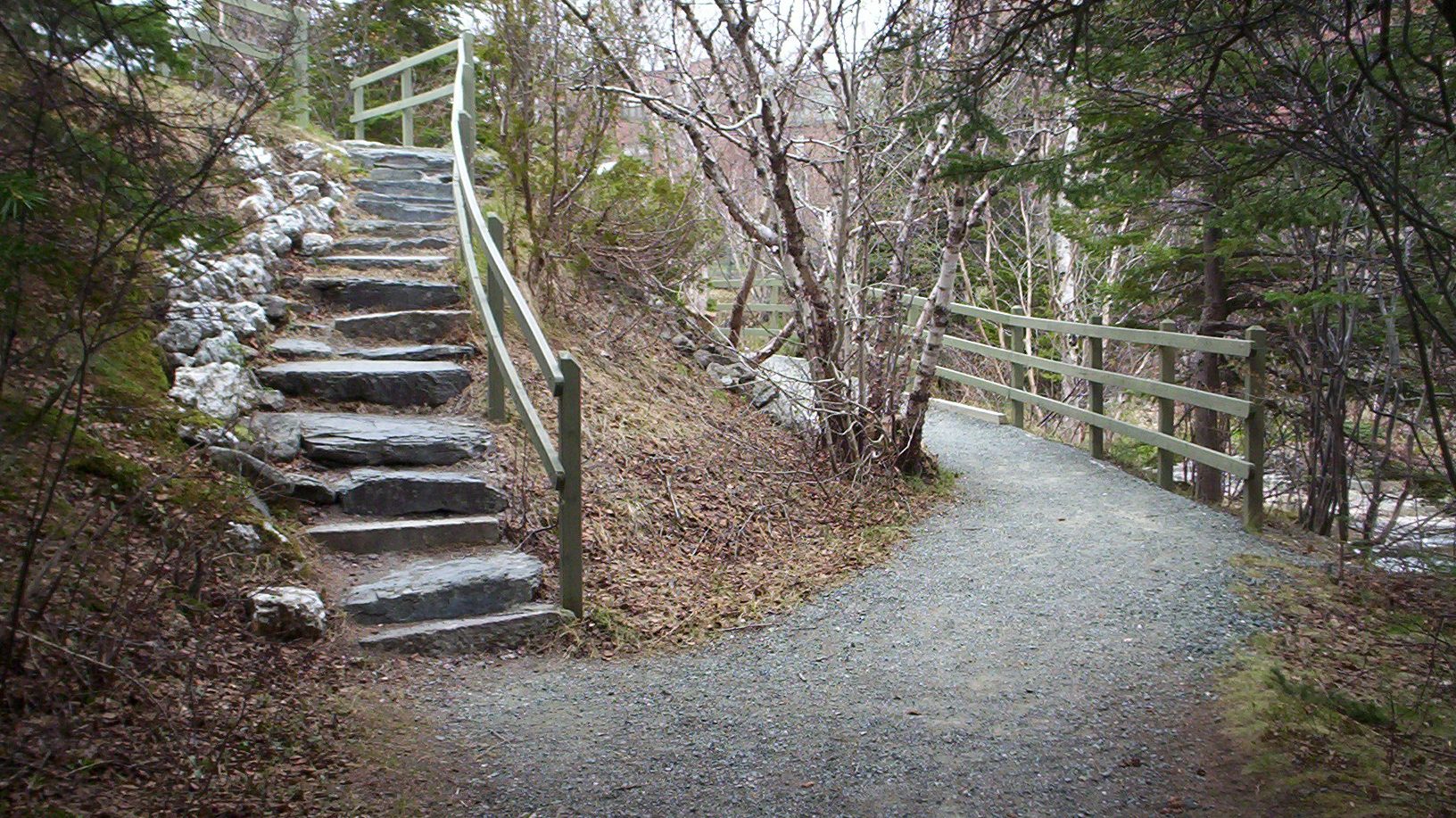
Consider taking another path
So, you have tried all the above but still, continue to wrestle bug after bug wasting your time? It might be time to rethink your tactic.
Maybe it’s time to look into a different method to achieve the result you want? It is okay to comment a big chunk of your code and start with a clean slate.
Open a new browser tab and look if there is someone else who tried to do the same thing before you. You now probably know what doesn’t work so you have one less method to try out and a better understanding of what you want to do.
Okay, so even this failed? Time to go back to school and read about the technology or libraries you are trying to use. You are most probably trying to do something unfit for the language or tech stack you initially chose. Going back to the drawing board might be smart in this case, as it gives you the chance to try a new way better fit for your project and saves you time otherwise wasted on forums.
Conclusion
You are now ready to hunt some wild bugs in your code but, most importantly, I hope that you took away some good practices to keep these pests away from your beautiful code. Of course, bugs always creep into your codes but knowing how to minimize their count and solve them in a quick fashion sets apart the experienced devs.
You will always remember that one bug that kept you up all night and depressed the next day. It is going to be a great trophy to add to your success collection. So don’t give up and hunt it down.
Happy coding and a good day!